Refund
If you want to return the funds to your shopper, you need to refund the payment.
You can refund either the full captured amount or a part of the captured amount. You can also perform multiple partial refunds, as long as their sum doesn't exceed the captured amount.
Some payment methods do not support partial refunds. To learn if a payment method supports partial refunds, refer to the payment method page such as cards, iDEAL, or Klarna.
Info
You can only refund a payment after it has already been captured.
Refund a payment
In order to refund a payment, you will need the transaction id from the transaction you want to refund. This identifier can be obtained in a few different ways:
- In the root of the create payment response message, you will find the field
transactionId
. This is an option if you have a server-to-server integration with Paybyrd . - For the hosted form integration, you can query the created order by its
orderId
and you may find the transactions associated to that order inside the nodeTransactions
. The fieldtransactionId
can be found there. - Whenever a payment is processed, Paybyrd will send you a notification in case you have it configured in your account. We will always send you the
transactionId
associated to that event. - You may also find the
transactionId
on Paybyrd's dashboard. Whenever you open the details of a payment, this identifier will be available under the namePayment Id
as shown below:
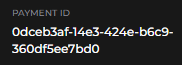
Payment ID - Dashboard
API Call
You can find below an example of how to refund a transaction and the generated response:
curl --request POST \
--url https://gateway.paybyrd.com/api/v2/refund/4faa13cd-f6ff-414e-b5bd-b61d1e72e418 \
--header 'Accept: application/json' \
--header 'Content-Type: application/json' \
--header 'x-api-key: 5E37D19C-F99C-445F-8B77-1463EFC66C7B' \
--data '{"amount":"10.00"}'
{
"sourceTransaction": {
"refundedAmount": "10.00",
"remainingAmount": "0.00",
"transactionId": "4faa13cd-f6ff-414e-b5bd-b61d1e72e418",
"amount": "10.00"
},
"code": "BYRD200",
"description": "Operation successfully completed",
"transactionId": "c16ce479-319d-4e7b-a966-7735c34b2cc5",
"amount": "10.00"
}
Handling rejections
The refund may not be approved for some reason and it's expected that you handle these scenarios on your side. Paybyrd will always respond with the code BYRD205
in case the refund cannot be done. Please see the example below:
curl --request POST \
--url https://gateway.paybyrd.com/api/v2/refund/4faa13cd-f6ff-414e-b5bd-b61d1e72e418 \
--header 'Accept: application/json' \
--header 'Content-Type: application/json' \
--header 'x-api-key: 5E37D19C-F99C-445F-8B77-1463EFC66C7B' \
--data '{"amount":"5.00"}'
{
"sourceTransaction": {
"refundedAmount": "0.00",
"remainingAmount": "5.00",
"transactionId": "4faa13cd-f6ff-414e-b5bd-b61d1e72e418",
"amount": "5.00"
},
"code": "BYRD205",
"description": "Operation rejected",
"transactionId": "c16ce479-319d-4e7b-a966-7735c34b2cc5",
"amount": "5.00"
}
Whenever you get this response code, you can make a new attempt later. BYRD205
means that the operation was declined by the bank, but the denial may change over the time. If you need more information why the refund was denied, please talk to our support team! \
Handling errors
In very exceptional scenarios, Paybyrd API may respond with errors. You can see below the most common responses. All the other cases will follow the same message structure:
{
"isError": true,
"code": "BYRD230",
"description": "The refund amount exceeds the remaining balance of the original payment"
}
{
"isError": true,
"code": "BYRD999",
"description": "Operation failed. This code is related to unhandled errors."
}
You can find all the possible codes returned by the Refund API here:
Code | Description | Transaction Status | Retryable |
---|---|---|---|
BYRD200 | Operation successfully completed | Success | No |
BYRD205 | Operation rejected | Denied | Yes |
BYRD230 | Payment already refunded | N/A | No |
BYRD231 | The refund amount exceeds the remaining balance of the original payment | N/A | No |
BYRD290 | An error occurred while pre processing the transaction. Please review your request and try again | Error | Yes |
BYRD291 | An error occurred during while preparing transaction for acquiring. Please review your request and try again | Error | Yes |
BYRD294 | Transaction could not be updated. Please review your request and try again | Error | Yes |
BYRD299 | Operation could not be completed. Please review your request and try again | Error | Yes |
BYRD403 | Resource access is forbidden. | Error | No |
BYRD401 | Resource access unauthorized. | Error | No |
BYRD900 | Invalid input. | Error | Yes |
BYRD901 | Resource not found. | Error | No |
BYRD999 | Operation failed. This code is related to unhandled errors. | Error | Yes |
BYRD010 | Request accepted | N/A | No |
See the full API reference here.
Updated over 1 year ago